Menu
Vojtěch Korduliak
Programmer and Photographer
Hidden Classes in JavaScript: Understanding V8's Performance Optimization
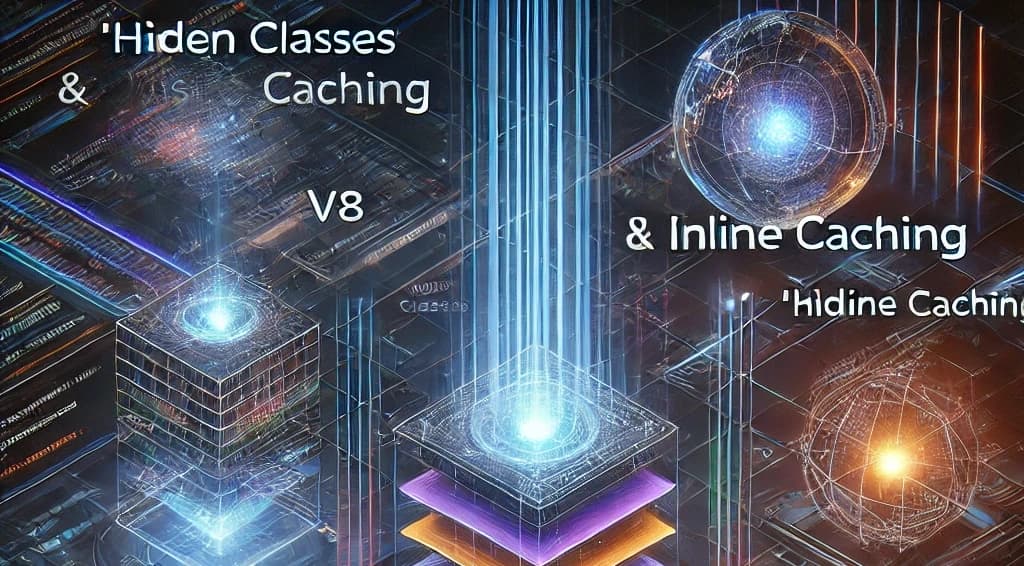
Have you ever wondered how your browser turns your JavaScript code into lightning-fast executions? Understanding these low-level mechanisms can be the key to optimizing your web application's performance. Let's dive in!
What is Inline Caching?
Inline caching is an optimization technique that speeds up access to object properties by caching their locations in memory. When an object's "shape" changes, the engine must invalidate this cache and recalculate property positions. Here's a practical example:
❌ Suboptimal approach:
const obj = {};
obj.name = 'John';
✅ Optimized approach:
const obj = { name: 'John' };
While this might seem like a minor difference, it becomes significant when dealing with larger objects and frequent property access patterns.
V8's Implementation of Inline Caching
V8 implements Inline Caching through Hidden Classes, a concept inspired by static languages like C++ and Java. In these languages, class layouts in memory are fixed, which means:
- All objects of the same class share an identical memory layout
- Each property has a predetermined offset in memory
- Property access occurs in constant time (O(1)) since the compiler knows exactly where to find each property
Back to JavaScript
JavaScript’s dynamic nature allows you to freely modify objects by adding, changing, or removing properties. While this flexibility is powerful, it can complicate optimization. To address these challenges, V8 uses “hidden classes”—virtual structures inspired by statically typed languages like C++ and Java. These hidden classes enable the engine to efficiently locate properties in memory without excessive computation.
So What Can You Do to Be More Effective?
A key strategy for improving performance is to keep your objects shapes as stable as possible. One way to do this is by initializing all properties early—either at object creation time or within a constructor. When you define properties immediately, the V8 engine can assign a single hidden class from the start. For example, creating an empty object and then adding properties one by one is less optimal than defining them all at once. By initializing everything up front, you avoid modifying the object’s shape later, which would otherwise force the engine to reconfigure its hidden classes.
Another tip is to maintain consistent property types. Changing a property’s data type (for instance, storing a string in a property that previously held a number) can prompt the engine to recompile or adjust internal optimizations. Different data types can have different memory requirements, so it’s more work behind the scenes. If a property is meant to hold a numerical value, stick to numbers instead of mixing in strings or objects.
Finally, it’s wise to avoid adding or removing properties after your object has been created. Each addition or removal requires generating or modifying hidden classes. If you need an optional property, consider setting it to null
or undefined
initially rather than creating it dynamically. This way, your object’s shape stays consistent and the engine can maintain its optimized structure.
Conclusion
Inline Caching is the primary way JavaScript engines optimize object property access, especially when object structures (shapes) change. In V8, this is managed with Hidden Classes, inspired by the statically defined classes in languages like C++ or Java. If your code frequently handles large numbers of objects or changes their structure often, keep in mind these optimizations:
- Initialize all properties when creating an object or in the constructor.
- Maintain consistent data types for each property.
- Measure and profile your application to confirm whether these micro-optimizations genuinely improve performance in your real-world scenario.
Remember: sometimes small optimizations can make a big difference, but in other cases, they may not be worth the extra complexity. Always let actual performance measurements guide your decisions.